4.1. Transforming Sequences with Comprehensions¶
Python has a very convenient method for describing one list in terms for another call a list comprehension. Comprehensions are very “functional” in their nature and we will make heavy use of these comprehensions moving forward.
4.1.1. List Comprehensions¶
Suppose that we wish to create a list from a sequence of values based on some selection criteria. An easy way to do this type of processing in Python is to use a list comprehension. List comprehensions are concise ways to create lists. The general syntax is:
[<expression> for <item> in <sequence> if <condition>]
where the if clause is optional. For example,
In [1]: mylist = [1,2,3,4,5]
In [2]: yourlist = [item ** 2 for item in mylist]
In [3]: yourlist
Out[3]: [1, 4, 9, 16, 25]
The expression describes each element of the list that is being built. The
for
clause iterates through each item in a sequence. The items are filtered
by the if
clause if there is one. In the example above, the for
statement lets item
take on all the values in the list mylist
one after
the other. Each item is then squared before it is added to the list that is
being built. The result is a list of squares of the values in mylist
.
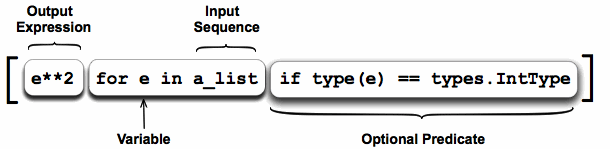
The built-in range
Python function is a useful tool for generating a
sequence of numbers. The sequence provided by range
always starts with 0.
If you ask for range(4)
, then you will get 4 values starting with 0. In
other words, 0, 1, 2, and finally 3. Notice that 4 is not included since we
started with 0. Likewise, range(10)
provides 10 values, 0 through 9.
In [4]: [ i for i in range(4)]
Out[4]: [0, 1, 2, 3]
In [5]: [x for x in range(10)]