7.3. Three General Tasks¶
When processing a sequence of data, problems can frequency be solved using a combination of three general operations: map, filter and reduce. Many of the examples from Chapter 4 can be framed in this light.
7.3.1. Map¶
A map is the operation of applying or mapping a function to each element of a list. An example would be squaring all the elements in a list.
In [1]: L = [1,2,3,4,5]
In [2]: sqr = lambda x: x**2
In [3]: sqr_L = [sqr(x) for x in L]
In [4]: sqr_L
Out[4]: [1, 4, 9, 16, 25]
In fact, the form of applying a map with a list comprehension will always have
the following form shown below for some function f
.
General Form of a map
mapped_L = [f(x) for x in L]
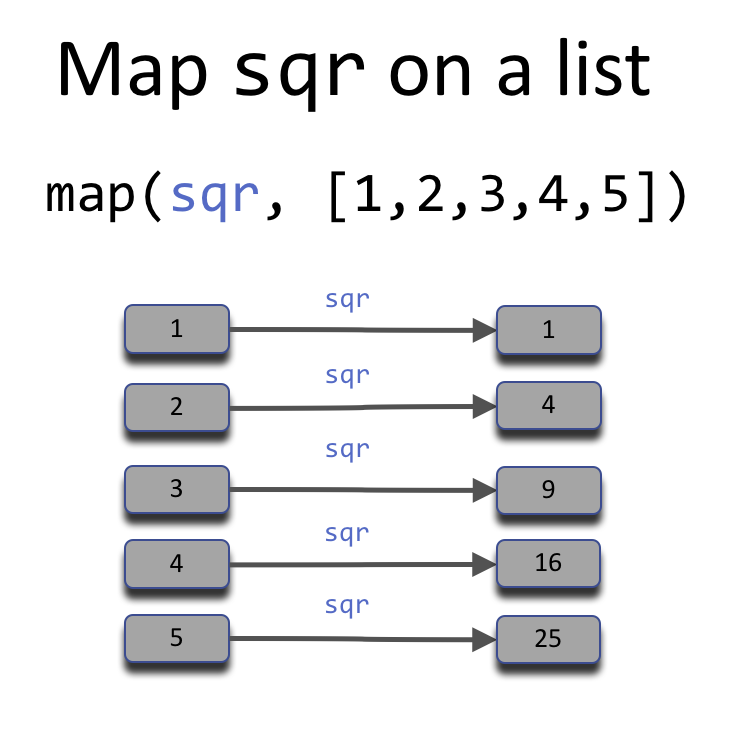
map
applies sqr
(first argument) to each element of the list (second argument).Since this is a common functional pattern, we should construct a general
abstraction. For this expression, we would want to be able to change the
function f
being mapped and the list L
. The following definition gives
an abstraction for map
(titled my_map
to avoid shadowing the built-in
map
).
In [5]: my_map = lambda func, L: [func(item) for item in L]
In [6]: my_map(lambda x: x**2, range(5))
Out[6]: [0, 1, 4, 9, 16]
In the above example, the first argument of my_map
is the function (lambda
expression) that will be applied to each element and the second argument is the
sequence to be processed. Python has a built-in function map
that
generalizes this process.
In [7]: out = map(lambda x: x**2, range(5))
In [8]: out
Out[8]: <map at 0x10fdb8390>
In [9]: type(out)