3.6. References to Sequences¶
In the next section, we will explore Python’s implementation of lists. In the process the idea of an alias is defined and illustrated, which will help explain some of the more confusing behaviors related to mutable objects such as lists.
3.6.1. Objects and References¶
If we execute these assignment statements,
a = "banana"
b = "banana"
we know that a
and b
will refer to a string with the letters
"banana"
. But we don’t know yet whether they point to the same string.
There are two possible ways the Python interpreter could arrange its internal states:
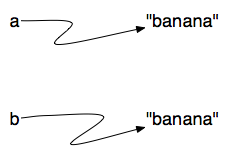
or
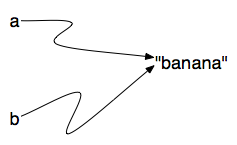
In one case, a
and b
refer to two different string objects that have the
same value. In the second case, they refer to the same object. Remember that an
object is something a variable can refer to.
We already know that objects can be identified using their unique identifier. We can also test whether two names refer to the same object using the is operator. The is operator will return true if the two references are to the same object. In other words, the references are the same. Try our example from above.
In [1]: a = "banana"
In [2]: b = "banana"
In [3]: a is b
Out[3]: True
The answer is True
. This tells us that both a
and b
refer to the
same object, and that it is the second of the two reference diagrams that
describes the relationship. Since strings are immutable, Python optimizes
resources by making two names that refer to the same string value refer to the
same object.
This is not the case with lists. Consider the following example. Here, a
and b
refer to two different lists, each of which happens to have the same
element values.
In [4]: a = [81, 82, 83]
In [5]: b = [81, 82, 83]
In [6]: a is b
Out[6]: False
In [7]: a == b